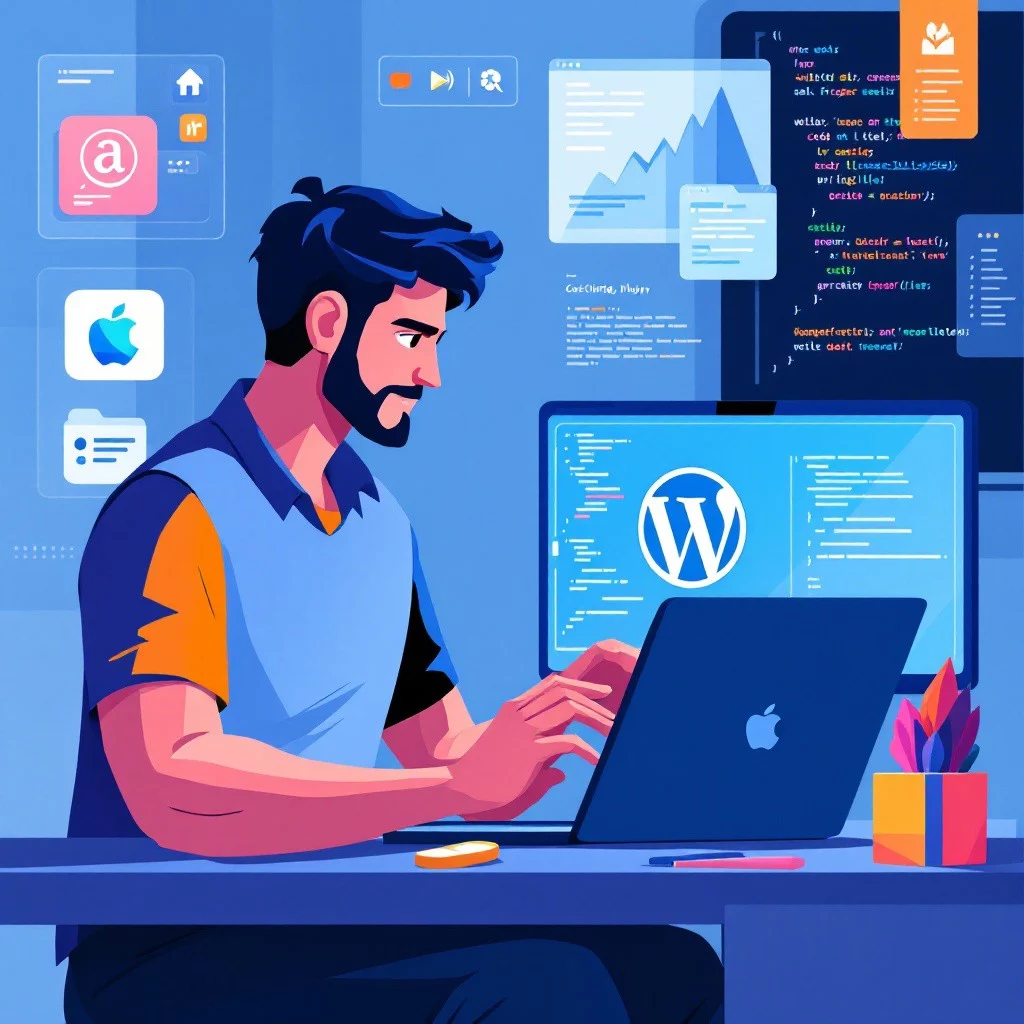
How to Write a Basic WordPress Plugin
So, you've been tinkering with WordPress and thought, "Hey, wouldn't it be cool if I could add my own features?" Well, you're in luck! Writing a basic WordPress plugin is not as daunting as it might seem. With a little bit of PHP knowledge and some patience, you'll be crafting custom plugins in no time. Let's dive into the process step by step.
Why Create a WordPress Plugin?
Before we get our hands dirty, let's chat about why you might want to create a plugin in the first place.
- Extend Functionality: Plugins let you add new features without modifying the core WordPress files.
- Reusable Code: You can use your plugin across multiple WordPress sites.
- Share with the Community: If your plugin solves a common problem, you can share it with others.
- Learning Experience: It's a great way to deepen your understanding of WordPress.
Getting Started
Prerequisites
- Basic Knowledge of PHP: You don't need to be a guru, but understanding PHP syntax is essential.
- Local Development Environment: Tools like XAMPP, WAMP, or Local by Flywheel can help you set up WordPress locally.
- A Text Editor: VSCode, Sublime Text, or even Notepad++ will do.
Understanding WordPress Plugin Structure
A WordPress plugin is essentially a PHP script with a specific header comment. WordPress recognizes this comment and makes your plugin available in the admin area.
Step-by-Step Guide
Step 1: Create a New Plugin Folder
Navigate to your WordPress installation directory:
/your-wordpress-site/wp-content/plugins/
Create a new folder for your plugin:
/your-wordpress-site/wp-content/plugins/my-first-plugin/
Step 2: Create the Main PHP File
Inside your plugin folder, create a PHP file with the same name:
/your-wordpress-site/wp-content/plugins/my-first-plugin/my-first-plugin.php
Step 3: Add Plugin Header Comment
Open my-first-plugin.php
and add the following header:
<?php
/**
* Plugin Name: My First Plugin
* Plugin URI: https://example.com/my-first-plugin
* Description: A simple plugin to display a custom message.
* Version: 1.0
* Author: Your Name
* Author URI: https://example.com
* License: GPL2
*/
This comment block tells WordPress about your plugin.
Step 4: Write Your Plugin Code
Let's make our plugin display a custom message at the end of each blog post.
Adding a Function
function mfp_custom_message($content) {
if (is_single()) {
$content .= '<p style="background-color: #eef; padding: 10px;">Thank you for reading!</p>';
}
return $content;
}
Hooking the Function
add_filter('the_content', 'mfp_custom_message');
Step 5: Activate the Plugin
- Go to your WordPress admin dashboard.
- Navigate to Plugins > Installed Plugins.
- Find My First Plugin in the list.
- Click Activate.
Visit a single blog post to see your custom message in action!
Enhancing the Plugin
Now that we've got a basic plugin running, let's make it a bit more dynamic.
Adding a Settings Page
We'll add a settings page where users can customize the message.
Step 1: Add Menu Item
add_action('admin_menu', 'mfp_add_admin_menu');
function mfp_add_admin_menu() {
add_options_page(
'My First Plugin Settings',
'My First Plugin',
'manage_options',
'my-first-plugin',
'mfp_options_page'
);
}
Step 2: Create Settings Page Callback
function mfp_options_page() {
?>
<div class="wrap">
<h1>My First Plugin Settings</h1>
<form action="options.php" method="post">
<?php
settings_fields('mfp_options_group');
do_settings_sections('my-first-plugin');
submit_button();
?>
</form>
</div>
<?php
}
Step 3: Register Settings
add_action('admin_init', 'mfp_settings_init');
function mfp_settings_init() {
register_setting('mfp_options_group', 'mfp_options');
add_settings_section(
'mfp_section_developers',
__('Custom Settings', 'my-first-plugin'),
'mfp_section_developers_callback',
'my-first-plugin'
);
add_settings_field(
'mfp_field_message',
__('Custom Message', 'my-first-plugin'),
'mfp_field_message_callback',
'my-first-plugin',
'mfp_section_developers'
);
}
function mfp_section_developers_callback() {
echo __('Set your custom message below:', 'my-first-plugin');
}
function mfp_field_message_callback() {
$options = get_option('mfp_options');
?>
<input type="text" name="mfp_options[mfp_field_message]" value="<?php echo isset($options['mfp_field_message']) ? esc_attr($options['mfp_field_message']) : ''; ?>">
<?php
}
Step 4: Update the Function to Use the Setting
function mfp_custom_message($content) {
if (is_single()) {
$options = get_option('mfp_options');
$message = isset($options['mfp_field_message']) ? $options['mfp_field_message'] : 'Thank you for reading!';
$content .= '<p style="background-color: #eef; padding: 10px;">' . esc_html($message) . '</p>';
}
return $content;
}
Now, users can set their own message via the WordPress admin dashboard!
Best Practices
Security Measures
- Sanitize User Input: Always sanitize and validate data.
- Use
sanitize_text_field()
,esc_html()
, etc.
- Use
- Escape Output: Before outputting data, escape it to prevent XSS attacks.
- Use
esc_attr()
,esc_url()
, etc.
- Use
- Nonces: Use WordPress nonces for form validation.
Coding Standards
- Follow WordPress Coding Standards: Makes your code readable and maintainable.
- Comment Your Code: Helps others (and future you) understand what the code does.
Internationalization (i18n)
- Wrap strings with translation functions:
__('Text', 'my-first-plugin')
for simple strings._e('Text', 'my-first-plugin')
when echoing.
Documentation
- Readme File: If you plan to distribute your plugin, include a
readme.txt
with detailed information. - Change Logs: Keep track of changes for each version.
Testing Your Plugin
- Debugging Mode: Enable
WP_DEBUG
inwp-config.php
. - Check for Errors: Look for PHP errors, warnings, and notices.
- Cross-Browser Testing: Ensure it works on all major browsers.
- Compatibility: Test with different themes and plugins.
Troubleshooting
Plugin Not Showing Up
- Check Header Comment: Ensure your plugin header is correct.
- File Permissions: Make sure your plugin files have the correct permissions.
Plugin Causes Errors
- PHP Syntax Errors: Check your code for typos or missing semicolons.
- Conflict with Other Plugins: Deactivate other plugins to isolate the issue.
Next Steps
- Add More Features: Like shortcode support or widget functionality.
- Submit to WordPress.org: Share your plugin with the community.
- Learn More: Explore the WordPress Plugin Handbook.
Conclusion
Congratulations! You've just created a basic WordPress plugin from scratch. Not only does this open up a world of possibilities for customizing your own site, but it's also a stepping stone towards becoming a seasoned WordPress developer.
Remember, the key to mastering plugin development is practice and continuous learning. Keep experimenting with new ideas, read up on best practices, and don't hesitate to seek help from the WordPress community.
Happy coding!